To use nuget.exe CLI commands in the console, see Using the nuget.exe CLI in the console. Find and install a package. For example, finding and installing a package is done with three easy steps: Step 1: Open the project/solution in Visual Studio, and open the console using the Tools NuGet Package Manager Package Manager Console command. The Ctrl + Tab could help us leave Package Manager Console window to other window, but it will not close it. If you want to close the Package Manager Console window, please use SHIFT + ESC to close current opened window in Visual Studio. I have tested in my Visual Studio 2015.
Like most developers, I prefer to not have to pick up the mouse if I don't have to. I use the Executor launcher for almost everything so it's extremely rare for me to ever click the 'Start' button in Windows. I also use shortcuts keys when I can so I don't have to pick up the mouse. By now most people know that the Package Manager Console that comes with NuGet is PowerShell embedded inside of Visual Studio. It is based on its PowerConsole predecessor which was the first (that I'm aware of) to embed PowerShell inside of Visual Studio and give access to the Visual Studio automation DTE object. It does this through an inherent $dte variable that is automatically available and ready for use. This variable is also available inside of the NuGet Package Manager console.
Adding a new class file to a Visual Studio project is one of those mundane tasks that should be easier. First I have to pick up the mouse. Then I have to right-click where I want it file to go and select 'Add –> New Item…' or 'Add –> Class…'
If you know the Ctrl+Shift+A shortcut, then you can avoid the mouse for adding a new item but you have to manually assign a shortcut for adding a new class. At this point it pops up a dialog just so I can enter the name of the class I want. Since this is one of the most common tasks developers do, I figure there has to be an easier way and a way that avoids picking up the mouse and popping up dialogs. This is where your embedded PowerShell prompt in Visual Studio comes in. The first thing you should do is to assign a keyboard shortcut so that you can get a PowerShell prompt (i.e., the Package Manager console) quickly without ever picking up the mouse. I assign 'Ctrl+P, Ctrl+M' because 'P + M' stands for '**P**ackage **M**anager' so it is easy to remember:
At this point I can type this command to add a new class:


which will result in the class being added:
At this point I've satisfied my original goal of not having to pick up a mouse and not having the 'Add New Item' dialog pop up. However, having to remember that $dte method call is not very user-friendly at all. The best thing to do is to make this a re-usable function that always loads when Visual Studio starts up. There is a $profile variable that you can use to figure out where that location is for your machine:
If the NuGet_profile.ps1 file does not already exist, you can just create it yourself and place it in the directory. Now you can put a function inside like this:
Since it's in the NuGet_profile.ps1 file, this function will automatically always be available for me after starting Visual Studio. Now I can simply do this:

At this point, we have a very nice developer experience. All I did to add a new class was: 'Ctrl-P, Ctrl-M', then 'addClass Foo'. No mouse, no pop up dialogs, no complex commands to remember. In fact, PowerShell gives you auto-completion as well. If I type 'addc' followed by [TAB], then intellisense pops up:
You can see my custom function appear in intellisense above. Now I can type the next letter 'c' and [TAB] to auto-complete the command. And if that's still too many key strokes for you, then you can create your own PowerShell custom alias for your function like this:
While all this is very useful, I did run into some issues which prompted me to make even further customization. This command will add the new class file to the current active directory. Depending on your context, this may not be what you want. For example, by convention all view model objects go in the 'Models' folder in an MVC project. So if the current document is in the Controllers folder, it will add your class to that folder which is not what you want. You want it to always add it to the 'Models' folder if you are adding a new model in an MVC project. For this situation, I added a new function called 'addModel' which looks like this:
First I figure out the path to the Models directory on line #7. Then I activate the Solution Explorer window on line #8. Then I make sure the Models directory is selected so that my context is correct when I add the new class and it will be added to the Models directory as desired.
These are just a couple of examples for things you can do with the PowerShell prompt that you have available in the Package Manager console. As developers we spend so much time in Visual Studio, why would you not customize it so that you can work in whatever way you want to work?! The next time you're not happy about the way Visual Studio makes you do a particular task – automate it! The sky is the limit.
-->Visual Studio Package Manager Console Mac
There are a number of situations, described below under When to Reinstall a Package, where references to a package might get broken within a Visual Studio project. In these cases, uninstalling and then reinstalling the same version of the package will restore those references to working order. Updating a package simply means installing an updated version, which often restores a package to working order.
In Visual Studio, the Package Manager Console provides many flexible options for updating and reinstalling packages.
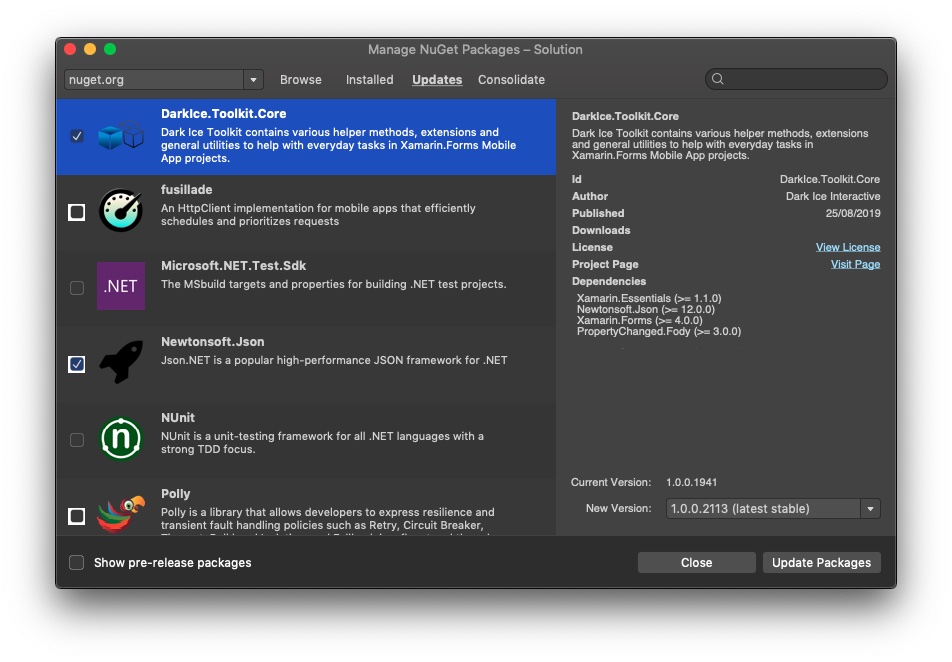
Updating and reinstalling packages is accomplished as follows:
Method | Update | Reinstall |
---|---|---|
Package Manager console (described in Using Update-Package) | Update-Package command | Update-Package -reinstall command |
Package Manager UI | On the Updates tab, select one or more packages and select Update | On the Installed tab, select a package, record its name, then select Uninstall. Switch to the Browse tab, search for the package name, select it, then select Install). |
nuget.exe CLI | nuget update command | For all packages, delete the package folder, then run nuget install . For a single package, delete the package folder and use nuget install <id> to reinstall the same one. |
Note
For the dotnet CLI, the equivalent procedure is not required. In a similar scenario, you can restore packages with the dotnet CLI.
In this article:
When to Reinstall a Package
Visual Studio Package Manager Console Add-migration
- Broken references after package restore: If you've opened a project and restored NuGet packages, but still see broken references, try reinstalling each of those packages.
- Project is broken due to deleted files: NuGet does not prevent you from removing items added from packages, so it's easy to inadvertently modify contents installed from a package and break your project. To restore the project, reinstall the affected packages.
- Package update broke the project: If an update to a package breaks a project, the failure is generally caused by a dependency package which may have also been updated. To restore the state of the dependency, reinstall that specific package.
- Project retargeting or upgrade: This can be useful when a project has been retargeted or upgraded and if the package requires reinstallation due to the change in target framework. NuGet shows a build error in such cases immediately after project retargeting, and subsequent build warnings let you know that the package may need to be reinstalled. For project upgrade, NuGet shows an error in the Project Upgrade Log.
- Reinstalling a package during its development: Package authors often need to reinstall the same version of package they're developing to test the behavior. The
Install-Package
command does not provide an option to force a reinstall, so useUpdate-Package -reinstall
instead.
Constraining upgrade versions
By default, reinstalling or updating a package always installs the latest version available from the package source.
In projects using the packages.config
management format, however, you can specifically constrain the version range. For example, if you know that your application works only with version 1.x of a package but not 2.0 and above, perhaps due to a major change in the package API, then you'd want to constrain upgrades to 1.x versions. This prevents accidental updates that would break the application.
To set a constraint, open packages.config
in a text editor, locate the dependency in question, and add the allowedVersions
attribute with a version range. For example, to constrain updates to version 1.x, set allowedVersions
to [1,2)
:
In all cases, use the notation described in Package versioning.
Using Update-Package
Being mindful of the Considerations described below, you can easily reinstall any package using the Update-Package command in the Visual Studio Package Manager Console (Tools > NuGet Package Manager > Package Manager Console).
Using this command is much easier than removing a package and then trying to locate the same package in the NuGet gallery with the same version. Note that the -Id
switch is optional.

The same command without -reinstall
updates a package to a newer version, if applicable. The command gives an error if the package in question is not already installed in a project; that is, Update-Package
does not install packages directly.
Visual Studio Package Manager Console Restore
By default, Update-Package
affects all projects in a solution. To limit the action to a specific project, use the -ProjectName
switch, using the name of the project as it appears in Solution Explorer:
To update all packages in a project (or reinstall using -reinstall
), use -ProjectName
without specifying any particular package:
To update all packages in a solution, just use Update-Package
by itself with no other arguments or switches. Use this form carefully, because it can take considerable time to perform all the updates:
Updating packages in a project or solution using PackageReference always updates to the latest version of the package (excluding pre-release packages). Projects that use packages.config
can, if desired, limit update versions as described below in Constraining upgrade versions.
For full details on the command, see the Update-Package reference.
Considerations
Visual Studio Package Manager Console Mac
The following may be affected when reinstalling a package:
Visual Studio Package Manager Console Update
Reinstalling packages according to project target framework retargeting
- In a simple case, just reinstalling a package using
Update-Package –reinstall <package_name>
works. A package that is installed against an old target framework gets uninstalled and the same package gets installed against the current target framework of the project. - In some cases, there may be a package that does not support the new target framework.
- If a package supports portable class libraries (PCLs) and the project is retargeted to a combination of platforms no longer supported by the package, references to the package will be missing after reinstalling.
- This can surface for packages you're using directly or for packages installed as dependencies. It's possible for the package you're using directly to support the new target framework while its dependency does not.
- If reinstalling packages after retargeting your application results in build or runtime errors, you may need to revert your target framework or search for alternative packages that properly support your new target framework.
- In a simple case, just reinstalling a package using
requireReinstallation attribute added in packages.config after project retargeting or upgrade
- If NuGet detects that packages were affected by retargeting or upgrading a project, it adds a
requireReinstallation='true'
attribute inpackages.config
to all affected package references. Because of this, each subsequent build in Visual Studio raises build warnings for those packages so you can remember to reinstall them.
- If NuGet detects that packages were affected by retargeting or upgrading a project, it adds a
Reinstalling packages with dependencies
Update-Package –reinstall
reinstalls the same version of the original package, but installs the latest version of dependencies unless specific version constraints are provided. This allows you to update only the dependencies as required to fix an issue. However, if this rolls a dependency back to an earlier version, you can useUpdate-Package <dependency_name>
to reinstall that one dependency without affecting the dependent package.Update-Package –reinstall <packageName> -ignoreDependencies
reinstalls the same version of the original package but does not reinstall dependencies. Use this when updating package dependencies might result in a broken state
Reinstalling packages when dependent versions are involved
- As explained above, reinstalling a package does not change versions of any other installed packages that depend on it. It's possible, then, that reinstalling a dependency could break the dependent package.